This tutorial is about integrating facebook into your
android
application.
Step 1: Registering Your
Facebook Application:
Go to facebook developer app site and Create the
application.
create new facebook application and fill out
all the information
needed. And select Native Android App.
And note down your facebook
App ID.
Step
2: Creating Facebook
Reference Project :
Once you are done with registering
your facebook
application, you need to download
facebook SDK and create
a new library project. This reference project will be used
to
compile your
actual project.
1. Download facebook android SDK from git repo-
sitories.
2. In your Eclipse goto File ⇒ Import ⇒ Existing Projects
into Workspace and select the facebook project you downloaded
from git repository.
sitories.
2. In your Eclipse goto File ⇒ Import ⇒ Existing Projects
into Workspace and select the facebook project you downloaded
from git repository.
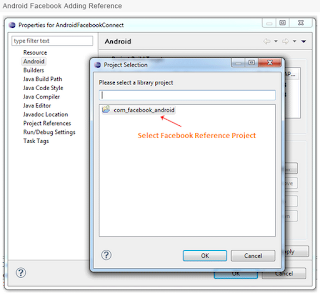
Now, You are ready to run the project :) .
Screen Shot of my project:
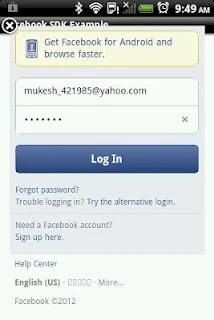
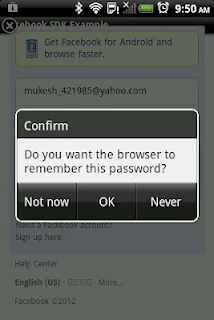
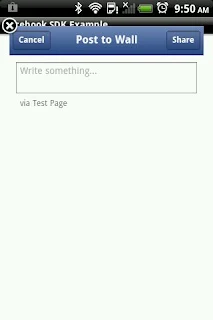
1. Get Profile Information:
/**
* Get Profile information by making request to Facebook Graph API
* */
public void getProfileInformation() {
mAsyncRunner.request("me", new RequestListener() {
@Override
public void onComplete(String response, Object state) {
Log.d("Profile", response);
String json = response;
try {
// Facebook Profile JSON data
JSONObject profile = new JSONObject(json);
// getting name of the user
final String name = profile.getString("name");
// getting email of the user
final String email = profile.getString("email");
// getting user name of the user
username = profile.getString("username");
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(getApplicationContext(), "Name: " + name + "\nEmail: " + email +"\nUserName : "+username, Toast.LENGTH_LONG).show();
}
});
} catch (JSONException e) {
e.printStackTrace();
}
}
@Override
public void onIOException(IOException e, Object state) {
}
@Override
public void onFileNotFoundException(FileNotFoundException e,
Object state) {
}
@Override
public void onMalformedURLException(MalformedURLException e,
Object state) {
}
@Override
public void onFacebookError(FacebookError e, Object state) {
}
});
}
2.Get All my Facebook Friends :
mAsyncRunner.request(username+"/picture", new RequestListener() {
@Override
public void onComplete(String response, Object state) {
Log.d("Profile", response);
String json = response;
try {
// Facebook Profile JSON data
JSONObject profile = new JSONObject(json);
JSONArray arrayFriend = profile.getJSONArray("data");
Log.d("Profile======", arrayFriend.toString());
// getting name of the user
if (arrayFriend != null) {
for (int i = 0; i < arrayFriend.length(); i++) {
String name = arrayFriend.getJSONObject(i).getString("name");
String id = arrayFriend.getJSONObject(i).getString("id");
Log.i(name,id);
}
}
final String name = profile.getString("name");
// getting email of the user
final String email = profile.getString("email");
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(getApplicationContext(), "Name: " + name + "\nEmail: " + email, Toast.LENGTH_LONG).show();
}
});
} catch (JSONException e) {
e.printStackTrace();
}
3. Getting my facebook profile Picture
Code:
ImageView user_picture;
user_picture=(ImageView)findViewById(R.id.imageView);
URL url;
try {
url = new URL("http://graph.facebook.com/"+username+"/picture?type=large");
Bitmap bmp = BitmapFactory.decodeStream(url.openConnection().getInputStream());
user_picture.setImageBitmap(bmp);
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
Hope , The above tutorial Helps
Anyone...Enjoy Coding... :)